Getting Started with Terraform: A Beginner's Guide to Basic Commands and Configuration
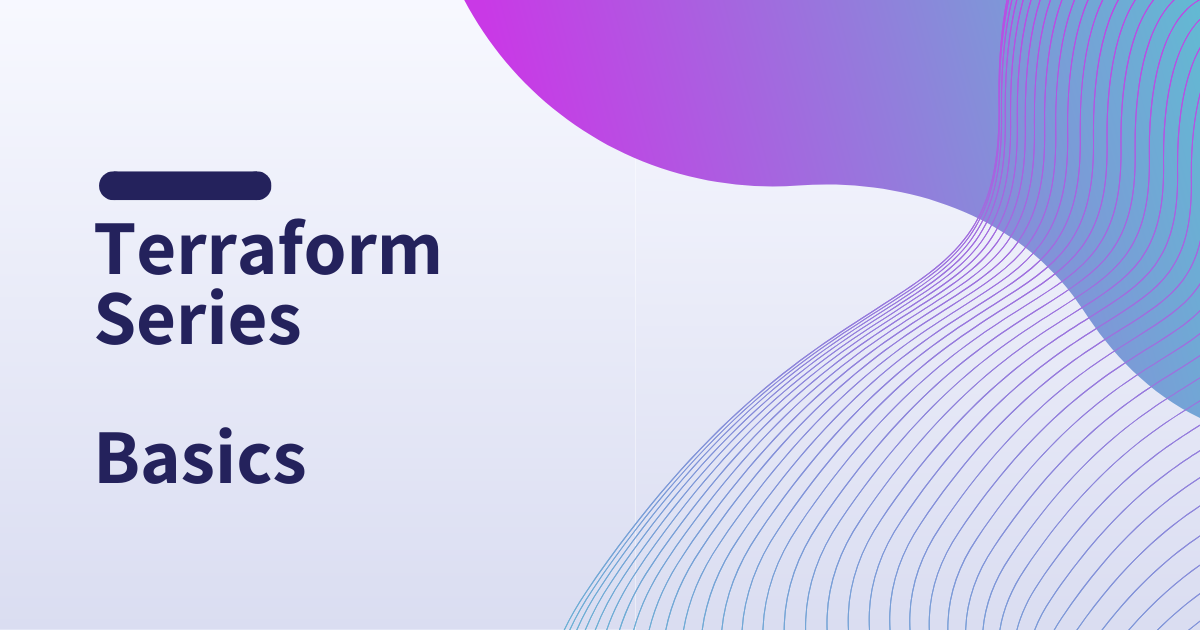
As more organizations embrace Infrastructure as Code (IaC), understanding Terraform's fundamentals becomes crucial. In this guide, I'll walk you through the essential Terraform commands, help you create your first configuration, and explain some key concepts that every DevOps engineer should know.
Essential Terraform Commands
The Holy Trinity of Terraform Commands
Let's start with the three commands you'll use most frequently in your Terraform journey.
1. terraform init: Your Starting Point
Think of terraform init
as the "npm install" or "pip install" of the Terraform world. It's always your first step when working with a new Terraform configuration or after cloning an existing one.
What it does:
- Initializes your working directory containing Terraform configuration files
- Downloads and installs required providers specified in your configuration
- Sets up the backend for storing Terraform's state file
- Downloads any modules referenced in your configuration
terraform init
Pro tip: Always run terraform init
after adding new providers or modules to your configuration.
2. terraform plan: Look Before You Leap
The terraform plan
command is your safety net. It shows you exactly what Terraform will do before it does it.
What it does:
- Reads the current state of any existing remote objects to make sure the state is up to date
- Compares the existing configuration with the previous state and notes any differences
- Proposes a set of changes needed to make your remote objects match the configuration
terraform plan
Pro tip: Use terraform plan -out=plan.tf
to save the plan to a file and ensure the exact same changes are applied later.
3. terraform apply: Making It Real
This is where the magic happens. terraform apply
takes your configuration and makes it reality.
What it does:
- By default, creates a new plan and asks for your confirmation
- Executes changes in the correct order, respecting resource dependencies
- Updates the state file with new resource details
- Displays any outputs defined in your configuration
terraform apply
# To skip the approval prompt:
terraform apply -auto-approve
Pro tip: While -auto-approve
is convenient for automation, always review the plan in production environments.
Creating Your First Terraform Configuration
Understanding main.tf
Let's look at a simple example using a local_file resource:
resource "local_file" "sample_resource" {
filename = "sample.txt"
content = "Hello, Terraform World!"
}
Breaking it down:
resource
: Tells Terraform you're defining a resource block"local_file"
: The resource type (provided by the local provider)"sample_resource"
: Your resource name (unique to your configuration)- Block parameters:
filename
: Where to create the filecontent
: What to write in the file
Project Structure Best Practices
A basic Terraform project structure looks like this:
your-project/
├── main.tf # Main configuration file
├── variables.tf # Variable declarations (optional)
├── outputs.tf # Output declarations (optional)
└── terraform.tfstate # State file (created after apply)
Step-by-Step Implementation
Let's create our first Terraform configuration:
- Create a new directory:
mkdir terraform-demo
cd terraform-demo
- Create main.tf:
# Create main.tf with your preferred editor
# Add the resource block from above
- Initialize Terraform:
terraform init
- Preview the changes:
terraform plan
Expected output will show:
- 1 resource to be added
- Details about the file to be created
- Apply the configuration:
terraform apply
After confirming with 'yes', Terraform will:
- Create the sample.txt file
- Write the content
- Update the state file
- Verify:
cat sample.txt
# Should output: Hello, Terraform World!
Additional Useful Commands
Here are some other commands you'll find helpful:
terraform destroy
: Removes all resources managed by your Terraform configuration
terraform destroy
terraform validate
: Checks if your configuration is valid
terraform validate
terraform show
: Displays the current state
terraform show
Understanding the Local Provider
The local_file resource we used in our example is part of the local provider, which manages local resources like files and directories.
Common properties for local_file:
filename
: (Required) Path where the file should be createdcontent
: (Optional) Content to be written to the filefile_permission
: (Optional) File permissions (e.g., "0644")directory_permission
: (Optional) Permissions for created parent directories (e.g., "0755")
State Management: The Heart of Terraform
Terraform tracks your managed infrastructure in a state file (terraform.tfstate). Think of it as Terraform's database of what it's managing.
The state file:
- Maps real-world resources to your configuration
- Tracks metadata
- Improves performance for large infrastructures
- Enables team collaboration
Best practices:
- Never edit the state file manually
- Always backup your state files
- Use remote state storage (S3, Azure Storage, etc.) for team environments
Final Thoughts
Understanding these basics sets a strong foundation for your Infrastructure as Code journey with Terraform. As you become more comfortable with these concepts, you'll be ready to tackle more complex configurations and manage real-world infrastructure.
Remember: Terraform is declarative - you describe what you want, and Terraform figures out how to make it happen. This makes it powerful but requires thinking differently about infrastructure management.
Stay tuned for more advanced topics like workspaces, remote state management, and working with multiple providers!